- The Composite Pattern is a Structural Design Pattern, it also known as partitioning design pattern. It describes a group of objects that is treated the same way as a single instance of the same type of object.
- It composes objects in terms of a tree structure
to represent part as well as whole hierarchy. Client treat individual objects
and compositions of objects uniformly.
Example:Consider you are creating Employee Management Application. Normally organization have hierarchy of CEO -> Director -> Managers -> Team Leader -> Developer. The owner of organization want to know all employee detail information. In normal application we have maintain lot of classes and subclass to get those of information. To overcome this problem composite pattern come to this place. It work as common interface component ((i.e.) IEmployee) and leaf tree node ((i.e.) Employee) and single full control composite class which is act as intermediator of component and leaf tree node.
UML Diagram:
Drawbacks:· It easy to add new kinds of components to your collection as long as they support similar programming interface.
· It’s harder to restrict the components of a composite.
Sample Demo Program:The following participant are involved in given program1. Component – It’s declare the interface for objects in the composition and for accessing and managing its child components. It also implement default behavior for the interface common to all classes as appropriate.2. Composite – It’s define components behaviors and implement child related operations ((i.e.) CRUD Operation) in the component interface3. Leaf – It’s defines behavior for primitive objects in the composition.4. Client – It’s manipulates the objects in the composition through the component interface.
Entire Code Structure:1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130
/** * Author : Manikandan M * Description: Sample program for composite pattern */ using System; using System.Collections.Generic; namespace CompositePattern { class Program { /// <summary> /// 'Component' Treenode /// </summary> interface IEmployee { void DisplayEmployeeDetails(); } /// <summary> /// 'Leaf' Class /// </summary> class Employee : IEmployee { private long empId; private string name; private string designation; private string experience; /// <summary> /// Get employee details with help of parameter constructor /// </summary> /// <param name="empId"></param> /// <param name="name"></param> /// <param name="designation"></param> /// <param name="experience"></param> public Employee(long empId, string name, string designation, string experience) { this.empId = empId; this.name = name; this.designation = designation; this.experience = experience; } /// <summary> /// Display employee details /// </summary> public void DisplayEmployeeDetails() { Console.WriteLine(string.Format("Emp Id: {0}, Employee Name: {1}, Designation: {2}, Experience: {3}\n", empId, name, designation, experience)); } } /// <summary> /// 'Composite' Class /// </summary> class Administrator : IEmployee { private List<IEmployee> employeeList = new List<IEmployee>(); /// <summary> /// Add new employee /// </summary> /// <param name="emp"></param> public void addEmployee(IEmployee emp) { employeeList.Add(emp); } /// <summary> /// Remove existing employee /// </summary> /// <param name="emp"></param> public void removeEmployee(IEmployee emp) { employeeList.Remove(emp); } /// <summary> /// display entire employee details /// </summary> public void DisplayEmployeeDetails() { foreach(IEmployee emp in employeeList) { emp.DisplayEmployeeDetails(); } } } //client or owner static void Main(string[] args) { Administrator administrator = new Administrator(); //add ceo information Employee ceo = new Employee(100, "Kumar", "CEO", "24 Years"); administrator.addEmployee(ceo); //add director information Employee hrDirector = new Employee(101, "Vimal", "HR Director", "20 Years"); Employee itDirector = new Employee(102, "Naveen", "IT Director", "18 Years"); Employee salesDirector = new Employee(103, "Pavan", "Sales Director", "22 Years"); administrator.addEmployee(hrDirector); administrator.addEmployee(itDirector); administrator.addEmployee(salesDirector); //add manager information Employee manager_1 = new Employee(104, "Ravi", "Manager", "15 Years"); Employee manager_2 = new Employee(105, "Mani", "Manager", "13 Years"); administrator.addEmployee(manager_1); administrator.addEmployee(manager_2); //add team leader information Employee teamleader_1 = new Employee(106, "Kannan", "Team Leader", "10 Years"); Employee teamleader_2 = new Employee(107, "Kumaran", "Team Leader", "8 Years"); administrator.addEmployee(teamleader_1); administrator.addEmployee(teamleader_2); //add developer information Employee developer_1 = new Employee(108, "Kathir", "Developer", "7 Years"); Employee developer_2 = new Employee(109, "Arun Pandiyan", "Developer", "5 Years"); administrator.addEmployee(developer_1); administrator.addEmployee(developer_2); administrator.DisplayEmployeeDetails(); Console.Write("Press any key to exist..."); Console.ReadKey(); } } }
Click here to download sample program
If you like this post. Kindly share your valuable comments.
Software Development Tricky
Friday, 6 October 2017
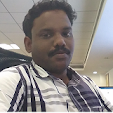
Composite Pattern
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment