- The Adapter Pattern is a Structural Design Pattern, its work as a bridge between two incompatible interfaces.
- It’s involves a single class which is responsible
to join functionalities of independent or incompatible interfaces.
Example:Consider you are creating video player application. First release of video player application can play AVI & WMV files only.After a while, your application becomes so popular that you get huge end user request to support other video file format as well.It’s good about product but how about the code? In this case you could rewrite your code to support other video format. To overcome this problem adapter pattern come to this place. It works as a bridge between two incompatible video formats ((i.e.) older video format & new video format which is expect from end users). It’s allows reusability of existing functionalities. It couldn’t modify existing functionality.
UML Diagram:
Drawbacks:
It’s harder to override Adaptee interface behaviour. It will require subclassing Adaptee and making Adapter refer to the subclass rather than the Adaptee Interface itself.
It unnecessarily increases the size of the code as class inheritance is less used and lot of code is needlessly duplicated between classes.
Sample Demo Program:
The following participants are involved in given program
1. Target Interface – This is the interface expected by the client
2. Adapter – This is a wrapper over Adaptee class which implements the Target Interface. It receives calls from the client and translates that request to one/ multiple adaptee calls using Adaptee Interface
3. Adaptee Interface – This is existing interface which is wrapped by Adapter. Client wants to interact with Adaptee but cannot interact directly because Adaptee interface is incompatible with Target Interface.
4. Client – Client will interact with Adapter using Target Interface
Entire Code Structure:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118
using System; namespace AdapterPattern { class Program { //'ITarget' Interface interface IVideoPlayer { void PlayVideo(string videoType, string fileName); } //'Adaptee' Interface which is act as additional feature interface interface IAdditionalVideoPlayer { void PlayFlv(string fileName); void PlayMpg(string fileName); } //'Adaptee' class 1 public class FlvPlayer : IAdditionalVideoPlayer { public void PlayFlv(string fileName) { Console.WriteLine("Playing flv File. Name: " + fileName); } public void PlayMpg(string fileName) { //do nothing } } //'Adaptee' class 2 public class MpgPlayer : IAdditionalVideoPlayer { public void PlayFlv(string fileName) { //do nothing } public void PlayMpg(string fileName) { Console.WriteLine("Playing mpg File. Name: " + fileName); } } //'Adapter' Class class VideoAdapter : IVideoPlayer { IAdditionalVideoPlayer additionalVideoPlayer; public VideoAdapter(string videoType) { if (videoType.Contains("flv")) { additionalVideoPlayer = new FlvPlayer(); } else if (videoType.Contains("mpg")) { additionalVideoPlayer = new MpgPlayer(); } } public void PlayVideo(string videoType, string fileName) { if (videoType.Contains("flv")) { additionalVideoPlayer.PlayFlv(fileName); } else if (videoType.Contains("mpg")) { additionalVideoPlayer.PlayMpg(fileName); } } } //'Target' class implementing the ITarget interface class VideoPlayer : IVideoPlayer { VideoAdapter videoAdapter; public void PlayVideo(string videoType, string fileName) { //inbuild support video files format if (videoType.Contains("avi") || videoType.Contains("wmv")) { Console.WriteLine(string.Format("Playing {0} file. Name: {1}", videoType, fileName)); } //videoAdapter is providing support to play other file formats else if (videoType.Contains("flv") || videoType.Contains("mpg")) { videoAdapter = new VideoAdapter(videoType); videoAdapter.PlayVideo(videoType, fileName); } else { Console.WriteLine("Invalid Video " + videoType + "file format not supported"); } } } //'Client' static void Main(string[] args) { VideoPlayer videoPlayer = new VideoPlayer(); Console.WriteLine("------------------------------------Adapter Pattern for Video Player-----------------------------\n"); videoPlayer.PlayVideo("avi", "bahubali-1.avi"); videoPlayer.PlayVideo("wmv", "bahubali-2.wmv"); videoPlayer.PlayVideo("flv", "wonder woman.flv"); videoPlayer.PlayVideo("mpg", "spider man.mpg"); Console.Write("Press any key to exist..."); Console.ReadKey(); } } }
Final Output:
Click here to download sample program
If you like this post. Kindly share your valuable comments
Software Development Tricky
Thursday, 28 September 2017
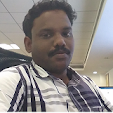
Adapter Pattern
Subscribe to:
Post Comments (Atom)
Wonderful article..
ReplyDelete